Tune in to the tools and techniques in the Elm ecosystem.
Similar Podcasts
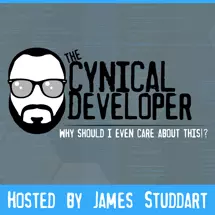
The Cynical Developer
A UK based Technology and Software Developer Podcast that helps you to improve your development knowledge and career,
through explaining the latest and greatest in development technology and providing you with what you need to succeed as a developer.
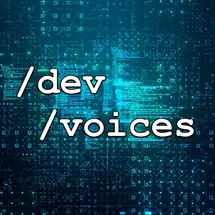
Developer Voices
Discover the future of software from the people making it happen.Listen to some of the smartest developers we know talk about what they're working on, how they're trying to move the industry forward, and what you can learn from it. You might find the solution to your next architectural headache, pick up a new programming language, or just hear some good war stories from the frontline of technology.Join your host Kris Jenkins as we try to figure out what tomorrow's computing will look like the best way we know how - by listening directly to the developers' voices.
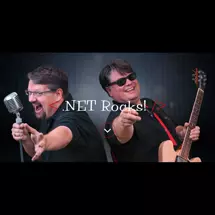
.NET Rocks!
.NET Rocks! is an Internet Audio Talk Show for Microsoft .NET Developers.
006: elm/parser
What is a parser?yacc/lexAST (Abstract Syntax Tree) vs. CST (Concrete Syntax Tree)JSON decoding vs. parsingJSON decoding is validating a data structure that has already been parsed. Assumes a valid structure.elm/parserHaskell parsec library - initially used for the Elm compiler, now uses custom parserWhat is a parser?One character at a timeTakes input string, turns it into structued data (or error)ComittingBacktrackable parserschompIf and chompWhileParser.oneOfBenchmarkingelm-explorations/benchmarkBenchmark before making assumptions about where performance bottlenecks areWrite unit tests for your parserGFM table parsing live streamParser.succeedElm regex vs elm parserIndications that you might be better off with parserLots of regex capture groupsWant very precise error messagesGetting source code locationsParser.getRow and getColParser.getSourceParser.loopLoop docs in elm/parserLooping allows you to track state and parse groups of expressionsLoop over repeated expression type, tell it termination condition with Step type (Loop and Done)Error MessagesYou can fail with Parser.problemParser.Advanced module is designed to give you more precise context and error messages on failureParser.Advanced.inContextGetting Started with a Parser ProjectWrite lots of unit tests with elm-test!There's likely a specification doc if you're parsing a language or formal syntaxCommonMark SpecGitHub-Flavored Markdown Specdillonkearns/elm-markdown test results directory from executing spec examplesLook at examples of parser projectsdillonkearns/elm-markdownelm-in-elm parserelm-in-elm conference talkmdgriffith/elm-markup - good reference for parsing, fault-tolerant parsing, and giving nice error messagesTereza's YAML parserTereza's elm conf talk "Demystifying Parsers"Jeroen's elm/parser Ellie"It's not hacking if you have tests."Look at elm/parser docs and resourceselm/parser project's semantics document describes backtrackable behavior in detail
005: How (And When) to Publish a Package
What is an Elm Package?Elm package repositoryElm packages enforce SemVer for the public package APIThe SemVer (Semantic Versioning) SpecInteresting note: the SemVer spec says breaking changes only refers to the public API. But a core contributor clarifies that breaking changes can come from changes to the public contract that you intend users to depend on. See this GitHub thread.list-extra packagedict-extra packageMinimize dependencies in your package to make it easier for users to manage their dependencies"Vendoring" elm packages (using a local copy instead of doing elm install author/package-name) can be useful in some cases to remove a dependency from your packageShould you publish a package?The Elm package ecosystem has a high signal to noise ratioElm packages always start at version 1.0.0SemVer has different semantics before 1.0.0 (patch releases can be breaking before 1) - see SemVer spec item 4Major version zero (0.y.z) is for initial development. Anything MAY change at any time. The public API SHOULD NOT be considered stable.Elm package philosophy is to do an alpha phase before publishing packageKeep yourself honest about solving meaningful problemsStart by asking "What problem are you solving? Who are you solving it for?"Scratch your own itchelm-graphqlservant-elm (for Haskell servant)Keep yourself honest about solving problems by starting with an examples/ folderEarly elm-graphql commits started with examples before anything elseWrite meaningful test cases with elm-testelm-verify-examplesHave a clear visionAsk people for feedbackLet ease of explanation guide you to refine core conceptsMake it easy for people to understand your package goals and philosophyInclude code examples in readme and docs to make it easier for people to get startedelm-review live stream videoUse meaningful examples solving concrete problems (images/screenshots are good, too)Richard Feldman's Exploring elm-spa-example talkLuke's elm-http-builder packageelm-spa-example's custom http request builder moduleInstead of writing a package, in some cases it could make sense to publish a blog post to share a patternPorting libraries vs. Coming Up With an Idiomatic Solution for ElmInstead of moment js ported to Elm, have an API built for a typed contextRyan's date-format packageHow to design an Elm package APIDefine your constraints/guarantees, make impossible states impossibleCharlie Koster's Advanced Types in Elm blog post seriesAvoid exposing internals of your dataElm Radio episode 002: Intro to Opaque TypesPay attention to how other packages solve problemsRichard Feldman's talk The Design Evolution of elm-css and elm-testBrian Hicks' talk Let's publish nice packagesLook at prior art, including in other ecosystemsLook at github issues and blog posts from projects in other ecosystemsPick your constraints instead of trying to solve every problemHelps you choose between tradeoffsHaving clear project goals explicitly in your Readme makes it easier to discuss tradeoffs with users and set expectationsIdiomatic elm package guide has lots of info on basic mechanics and best practices for publishing Elm packagesThe mechanics of publishing an elm packageelm make --docs docs.json will tell you if you're missing docs or if there are documentation validation errorselm-doc-previewCan use elm-doc-preview site to share documentation of branches, or packages that haven't been published yetSet up a CIDillon's CI script for dillonkearns/elm-markdown packageDillon's elm-publish-action GitHub Action will publish package versions when you increment the version in your elm.json - nice because it runs CI checks before finalizing a new package releaseelm publish will walk you through the steps you need before publishing the first version of your Elm package#packages channel on the Elm slack shows a feed of packages as they're published#api-design channel on the Elm slack is a good place to ask for feedback on your API design and package ideaContinue the ConversationShare your package ideas with us @elmradiopodcast on Twitter!
004: JSON Decoders
Basicselm/json package docsElm Guide section on JSON DecodersValidates that data has the expected shape. Similar to the pattern we discussed in episode 002 Intro to Opaque Types.Ports and FlagsHere's an Ellie example that shows the error when you have an implicit type that your flags decode to and it's incorrect.Sorry Dillon... Jeroen won the trivia challenge this time 😉 It turns out that ports will throw exceptions when they are given a bad value from JavaScript, but it doesn't bring down your Elm app. Elm continues to run with an unhandled exception. Here's an Ellie example.Flags and ports will never throw a runtime exception in your Elm app if you always use Json.Decode.Values and Json.Encode.Values for them and handle unexpected cases. Flags and ports are the one place that Elm lets you make unsafe assumptions about JSON data.Benefits of Elm's Approach to JSONBad data won't end up deep in your application logic where it's hard to debug (and discover in the first place)Decouples serialization format from your Elm data typesYou can do data transformations locally as you build up your decoder, rather than passing your giant data structure through a single transform functionDecoding Into Ideal TypesRichard Feldman's elm-iso8601 packageelm/time packageProgramming by intentionJson.Decode.succeed is helpful for stubbing out dataDillon's Incremental Type Driven Design talk at elm EuropeJson.Decode.fail lets you validate data and fail your whole decoder if there’s a problemParse, don’t validate blog post by Alexis KingDillon's elm-cli-options-parser packageJson.Decode.maybe docsNote about Decode.maybe. It can be unsafe to use this function because it can cover up failures. Json.Decode.maybe will cover up some cases that you may not have intended to. For example, if an API returns a float we would suddenly get Nothing back, but we probably want a decoding failure here:import Json.Decode as Decode """ {"temperatureInF": 86} """ |> Decode.decodeString (Decode.maybe (Decode.field "temperatureInF" Decode.int)) --> Ok (Just 86) """ {"temperatureInF": 86.14} """ |> Decode.decodeString (Decode.maybe (Decode.field "temperatureInF" Decode.int)) --> Ok Nothing Json.Decode.Extra.optionalNullableField might have more intuitive and desirable behavior for these cases.Thank you to lydell for the tip! See the discussion in this discourse thread.Learning resourceBrian Hicks' book The JSON Survival KitJoël Quenneville’s Blog Posts About JSON DecodersGetting Unstuck with Elm JSON Decoders5 Common JSON DecodersElm's Universal PatternGuaranteeing that json is valid before runtimeelm-graphql packageThe basics of GraphQLDillon's Types Without Borders Elm Conf talkElm Radio 001 Getting Started with elm-pageselm-pages StaticHttp API docsKris Jenkins' elm-export tool for Haskell types to Elm.Mario Rogic's Evergreen Elm elm Europe talkAutogenerating json decodersjson-to-elm tool - generates Elm decoders from raw JSON valuesintellij-elm JSON decoder generatorOrganizing your decodersEvan Czaplicki's elm Europe keynote The life of a fileEvan's experience report on implicit decoding in HaskellGetting StartedUnderstand Json.Decode.mapUnderstand record type aliases - the function that comes from defining type alias Album = { ... }Submit your question to Elm Radio!
003: Getting started with elm-review
elm-reviewreview-rgb-ranges package by RunarElm Analyseelm-review 1.0 release announcement blog postelm-review 2.0 release announcement blog postCustom Scalars in elm-graphql
002: Intro to Opaque Types
Opaque TypesSome patternsRuntime validations - conditionally return type, wrapped in Result or MaybeGuarantee constraints through the exposed API of the module (like PositiveInteger or AuthToken examples)Package-Opaque ModulesExample - the Element type in elm-ui.Definition of the Element type aliaselm-ui's elm.json file does not expose the internal module where the real Element type is defined.Example from elm-graphql codebase - CamelCaseName opaque type
001: Getting started with elm-pages
elm-pages hydrates into a full Elm app. It solves similar problems to what GatsbyJS solves in the ReactJS ecosystem.Static site generators with JS-free outputhttps://korban.net/elm/elmstatic/https://jekyllrb.com/EleventyMeta TagsOpen Graph tagsAsset management with elm-pages (CSS vs. SASS,etc.)Github issue discussing using the Unix Toolchain Philosophy in the context of keeping elm-pages focused on primitive assets for elm appsCompared to extending the Gatsby webpack configSOLID Open-Closed Principleelm-pages showcaseChandu's art showcase (built with elm-pages) - https://tennety.art/Headless CMSes vs. monolothic site providershttps://www.sanity.io/contentful.comhttps://airtable.com/netlifycms.orgCDN hosting provider NetlifyStatic Site Generators and The JAMstackhttps://jekyllrb.com/ - static site builder in Ruby - perhaps the first static site generator?Eleventy - spritual successor to Jekyll - but more flexibleMore info on what exactly is the JAMstack?Getting started with elm-pageselm-pages-starter repoelm-pages vs. elm/browserPages.Platform.applicationThe elm-pages StaticHttp APIStaticHttp Docs (there's a description of when and why you would use this compared to elm/http)elm-pages.com blog post A is for API - talks about StaticHttp and its lifecycle, including some example code.Core ConceptsSEO - elm-pages SEO API docsSecrets - docsSection in StaticHttp blog post about how you don't use Msgs for your StaticHttp datagenerateFiles hookIncremental Elm Live - Twitch streaming seriesWhere to learn moreelm-pages.comJoin the Elm slack and say hello in the elm-pages channel!